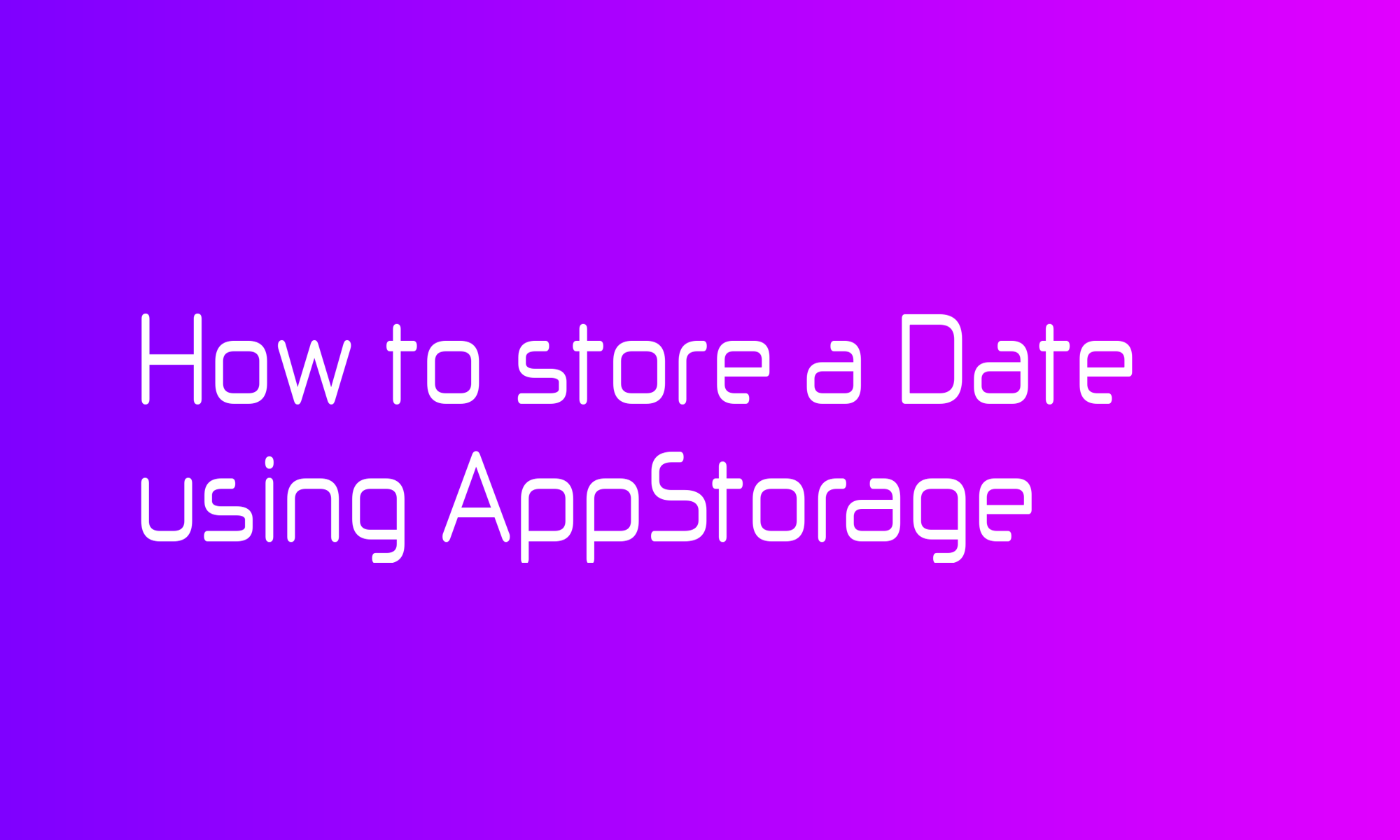
By default, @AppStorage
property wrapper supports Int
, String
, Double
, Data
, or URL
values. You can store other classes/structs/enums if you make them conform to the RawRepresentable
protocol. This tutorial will learn how to keep Dates in UserDefaults
handled by @AppStorage
.
If you write something like this:
@AppStorage("savedDate") var date: Date = Date()
the Swift compiler gives you an error because the @AppStorage
property wrapper doesn’t support Date type.
But it supports objects that conform to RawRepresentable
protocol, where that raw value is a String or Int. A proposed implementation may look like this:
import Foundation
extension Date: RawRepresentable {
private static let formatter = ISO8601DateFormatter()
public var rawValue: String {
Date.formatter.string(from: self)
}
public init?(rawValue: String) {
self = Date.formatter.date(from: rawValue) ?? Date()
}
}
This code uses ISO8601DateFormatter
to format a date to String and map it back. That formatter is static because creating and removing DateFormatters
is an expensive operation. If you add the code above to your project, you will be able to read and store dates in your SwiftUI app.
struct DateView: View {
@AppStorage("savedDate") var date: Date = Date()
var body: some View {
VStack {
Text(date, style: .date)
Text(date, style: .time)
Button("Save date") { date = Date() }
}
}
}
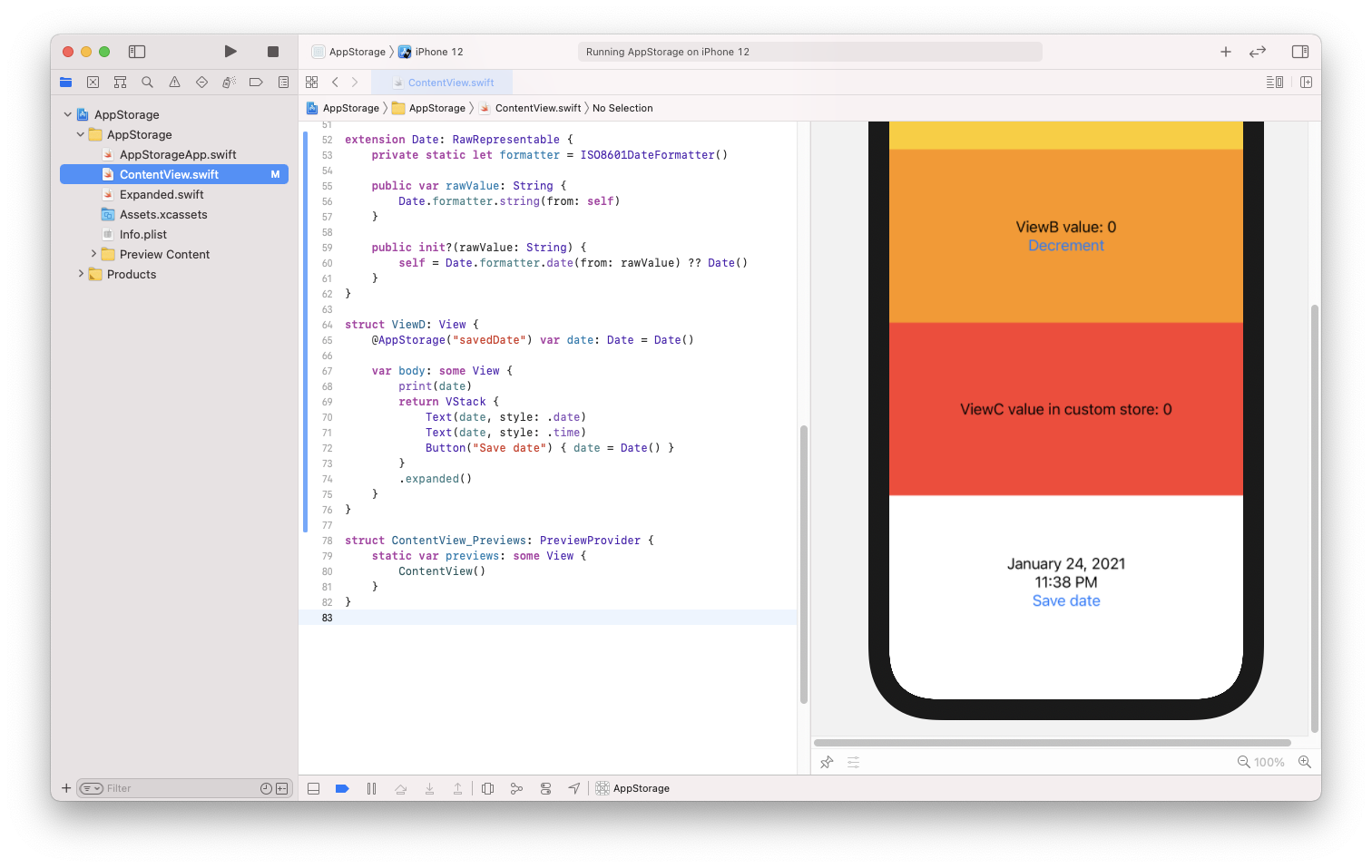