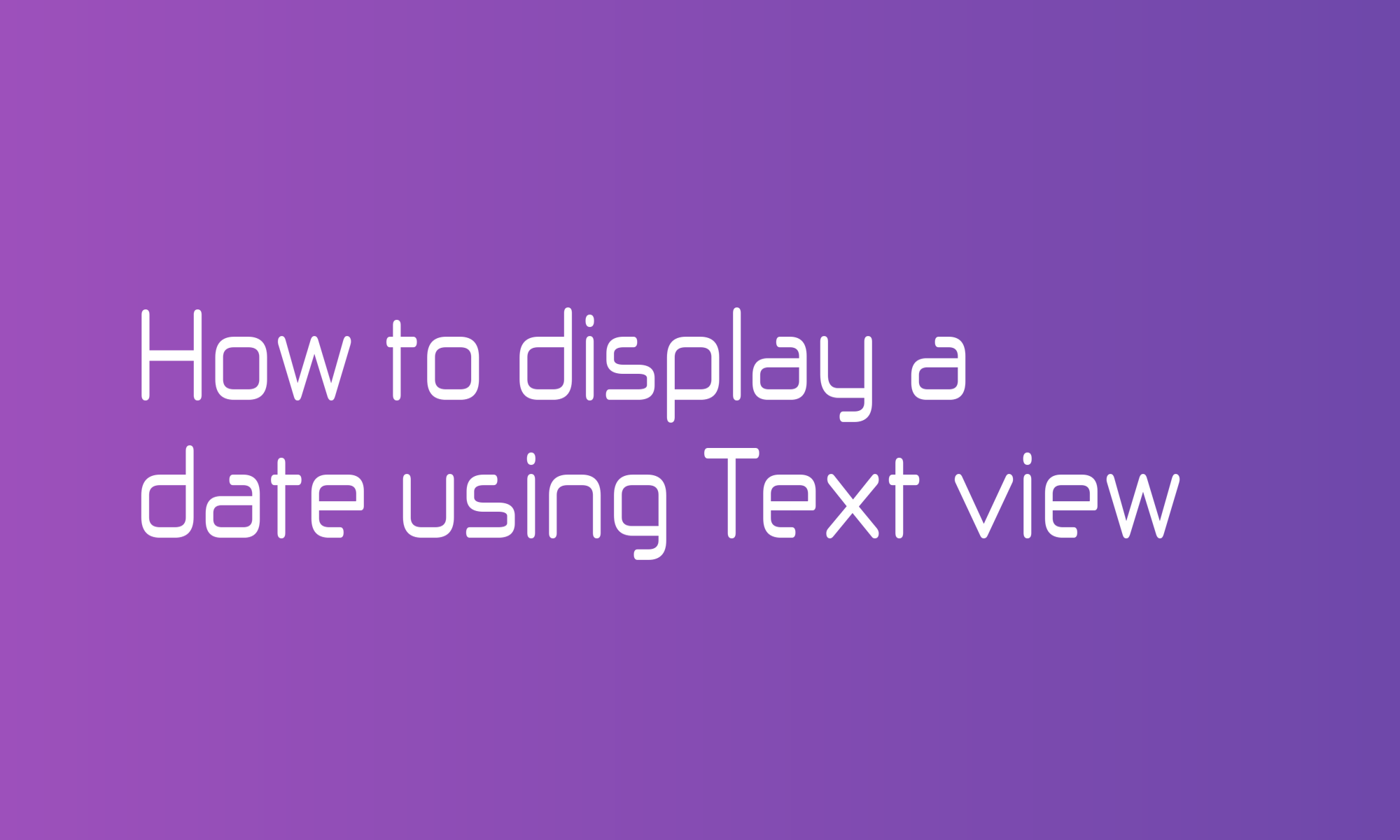
SwiftUI has a very convenient way to display dates. It provides a special Text initializer that receives date and style. You can pick from a list of five available DateStyles: time
, date
, relative
, offset
, and timer
. Each one displays time in a different format.
let now = Date() // Dec 6, 2020 at 2:51 PM
let twoDays: TimeInterval = 2 * 24 * 60 * 60
let date = now.advanced(by: twoDays) // Dec 8, 2020 at 2:51 PM
struct ContentView: View {
var body: some View {
VStack {
Text(date, style: .time) // 2:51 PM
Text(date, style: .date) // December 8, 2020
Text(date, style: .relative) // 1 day, 23 hr
Text(date, style: .offset) // -1 day
Text(date, style: .timer) // 47:59:50
}
}
}
Three date styles: relative
, offset
, and timer
, are dynamic styles and can display values that change over time. There are very useful if you want to create a widget using WidgetKit that counts down a time. Currently, this is the only way to display non-static elements in system widgets.
Two additional initializers handle ranges of date. They support DateIntervals and ClosedRange of dates. These two works very similar and you can use the one that’s suits you best. As you can see in the example below, they modify its looks based on the given dates.
let now = Date() // Dec 6, 2020 at 2:51 PM
let hundredMinutes: TimeInterval = 100 * 60
let twoDays: TimeInterval = 2 * 24 * 60 * 60
let date = now.advanced(by: twoDays) // Dec 8, 2020 at 2:51 PM
struct ContentView: View {
var body: some View {
VStack {
Text(DateInterval(start: now, duration: twoDays)) // Dec 6 - Dec 8
Text(DateInterval(start: now, duration: hundredMinutes)) // 2:51-4:31 PM
Text(now...date) // Dec 6 - Dec 8
}
}
}
You can use Swift string interpolation to display DateStyle text inside other strings. You will find that useful when you want to put your date in the middle of another sentence.
let now = Date() // Dec 6, 2020 at 2:51 PM
let twoDays: TimeInterval = 2 * 24 * 60 * 60
let date = now.advanced(by: twoDays) // Dec 8, 2020 at 2:51 PM
struct ContentView: View {
var body: some View {
Text("Styles can be used inside other strings with string interpolation: \(date, style: .relative)")
// Output: Styles can be used inside other strings with string interpolation: 1 day, 23 hr
}
}
Unfortunately, not all DateStyles are localized. Offset and relative always display its value in English, no matter what language your project supports.
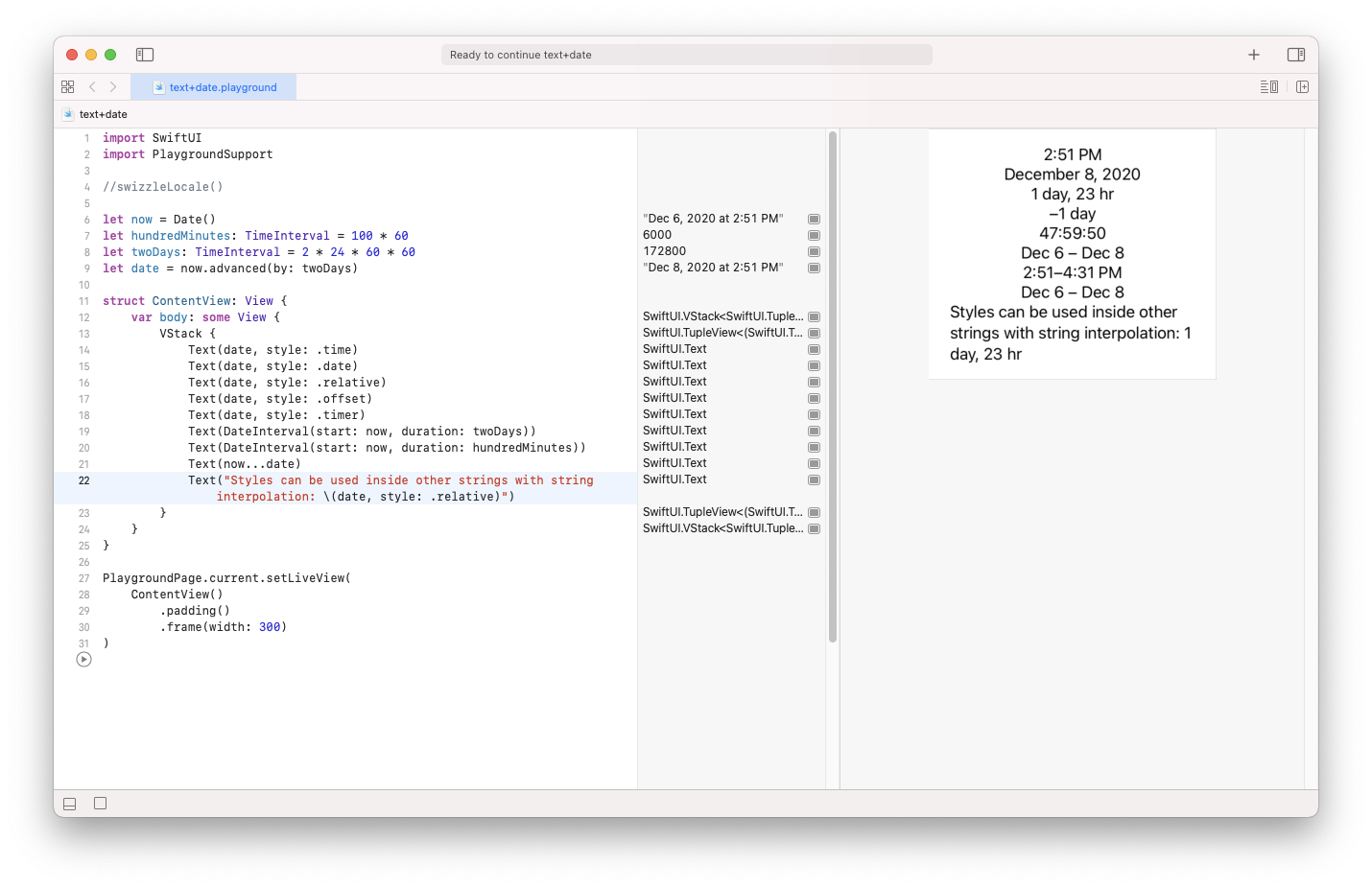